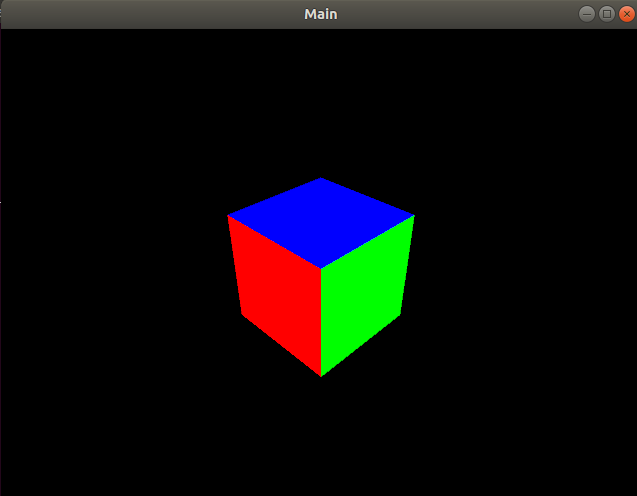
Pangolin: 2 - Use threads
# Targets
- Run pangolin in a seperate thread
<--more-->
# Source code
```cpp
#include
#include
#include <pangolin/pangolin.h>
static const std::string window_name = "Main";
static const int w = 640;
static const int h = 480;
void setup()
{
// create a window and bind its context to the main thread
pangolin::CreateWindowAndBind(window_name, w, h);
// enable depth
glEnable(GL_DEPTH_TEST);
// unset the current context from the main thread
pangolin::GetBoundWindow()->RemoveCurrent();
}
void run()
{
// fetch the context and bind it to this thread
pangolin::BindToContext(window_name);
// we manually need to restore the properties of the context
glEnable(GL_DEPTH_TEST);
// Define Projection and initial ModelView matrix
pangolin::OpenGlRenderState s_cam(
pangolin::ProjectionMatrix(w, h, 420, 420, 320, 240, 0.2, 100),
pangolin::ModelViewLookAt(-2, 2, -2, 0, 0, 0, pangolin::AxisY));
// Create Interactive View in window
pangolin::Handler3D handler(s_cam);
pangolin::View& d_cam = pangolin::CreateDisplay()
.SetBounds(0.0, 1.0, 0.0, 1.0, -float(w) / float(h))
.SetHandler(&handler);
while (!pangolin::ShouldQuit()) {
// Clear screen and activate view to render into
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
d_cam.Activate(s_cam);
// Render OpenGL Cube
pangolin::glDrawColouredCube();
// Swap frames and Process Events
pangolin::FinishFrame();
}
// unset the current context from the main thread
pangolin::GetBoundWindow()->RemoveCurrent();
}
int main(int argc, char** argv)
{
setup();
// use the context in a separate rendering thread
std::thread render_loop;
render_loop = std::thread(run);
render_loop.join();
return 0;
}
```
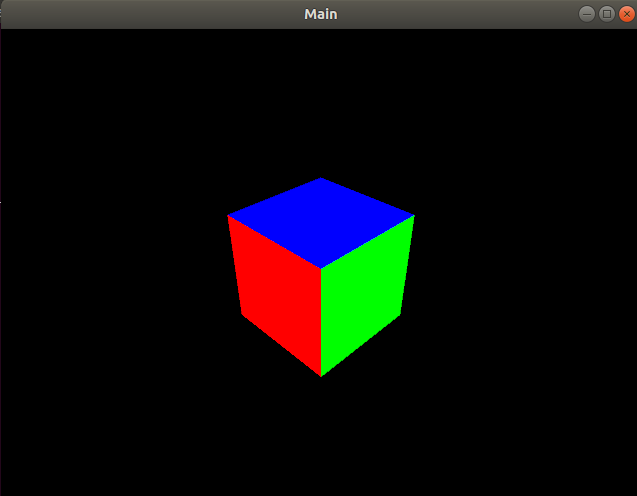
No comments