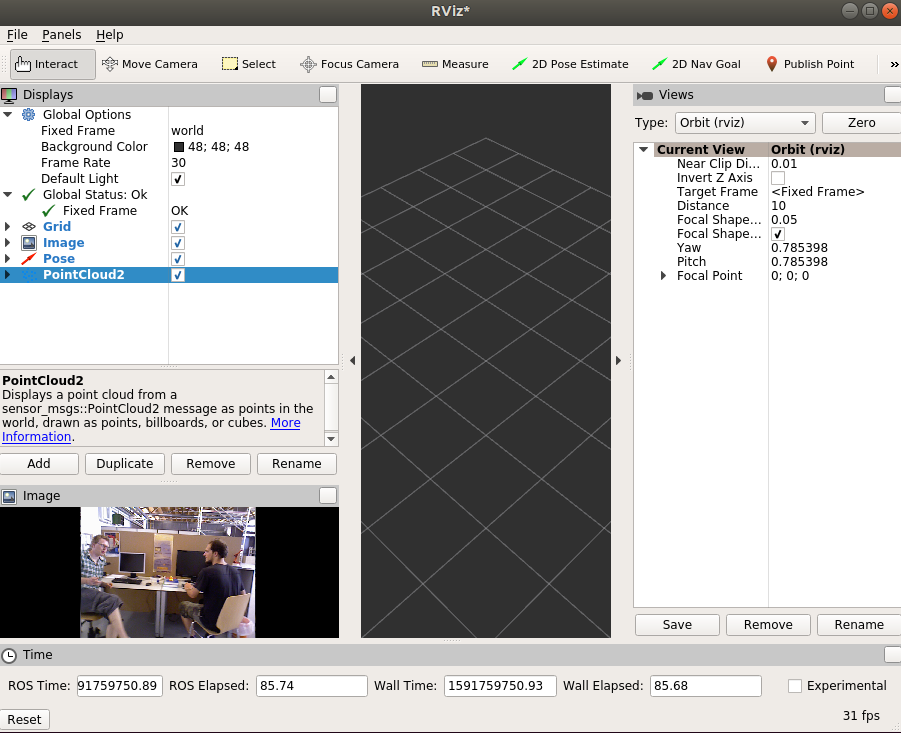
Docker: [Error] libGL error: failed to load driver: swrast
# Environment
- Host: ubuntu 18.04 with cuda 10.2 and nvidia-440
- Docker image: ubuntu 16.04
# Example: Run Rviz
```sh
# rosrun rviz rviz
[ INFO] [1591698594.187146454]: rviz version 1.12.17
[ INFO] [1591698594.187204754]: compiled against Qt version 5.5.1
[ INFO] [1591698594.187226653]: compiled against OGRE version 1.9.0 (Ghadamon)
libGL error: No matching fbConfigs or visuals found
libGL error: failed to load driver: swrast
Could not initialize OpenGL for RasterGLSurface, reverting to RasterSurface.
libGL error: No matching fbConfigs or visuals found
libGL error: failed to load driver: swrast
Segmentation fault (core dumped)
```
# Example: error when run OpenGL
```sh
$ sudo apt-get update
$ sudo apt-get install libglu1-mesa-dev freeglut3-dev mesa-common-dev
```
```cpp
#include
void displayMe(void)
{
glClear(GL_COLOR_BUFFER_BIT);
glBegin(GL_POLYGON);
glVertex3f(0.5, 0.0, 0.5);
glVertex3f(0.5, 0.0, 0.0);
glVertex3f(0.0, 0.5, 0.0);
glVertex3f(0.0, 0.0, 0.5);
glEnd();
glFlush();
}
int main(int argc, char** argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE);
glutInitWindowSize(400, 300);
glutInitWindowPosition(100, 100);
glutCreateWindow("Hello world!");
glutDisplayFunc(displayMe);
glutMainLoop();
return 0;
}
```
Compile:
```sh
g++ main.cpp -o firstOpenGlApp -lglut -lGLU -lGL
./firstOpenGlApp
```
Error detail:
```sh
# ./firstOpenGlApp
libGL error: No matching fbConfigs or visuals found
libGL error: failed to load driver: swrast
freeglut (./firstOpenGlApp): ERROR: Internal error in function fgOpenWindow
```
# Analysis
- locate
```sh
# locate libGL.so
/usr/lib/x86_64-linux-gnu/libGL.so
/usr/lib/x86_64-linux-gnu/mesa/libGL.so
/usr/lib/x86_64-linux-gnu/mesa/libGL.so.1
/usr/lib/x86_64-linux-gnu/mesa/libGL.so.1.2.0
```
- ldd
```sh
# ldd firstOpenGlApp
linux-vdso.so.1 => (0x00007fff839cf000)
libglut.so.3 => /usr/lib/x86_64-linux-gnu/libglut.so.3 (0x00007f0388eea000)
libGL.so.1 => /usr/lib/x86_64-linux-gnu/mesa/libGL.so.1 (0x00007f0388c76000)
libc.so.6 => /lib/x86_64-linux-gnu/libc.so.6 (0x00007f03888ac000)
libX11.so.6 => /usr/lib/x86_64-linux-gnu/libX11.so.6 (0x00007f0388572000)
libm.so.6 => /lib/x86_64-linux-gnu/libm.so.6 (0x00007f0388269000)
libXi.so.6 => /usr/lib/x86_64-linux-gnu/libXi.so.6 (0x00007f0388059000)
libXxf86vm.so.1 => /usr/lib/x86_64-linux-gnu/libXxf86vm.so.1 (0x00007f0387e53000)
libz.so.1 => /lib/x86_64-linux-gnu/libz.so.1 (0x00007f0387c39000)
libexpat.so.1 => /lib/x86_64-linux-gnu/libexpat.so.1 (0x00007f0387a10000)
libxcb-dri3.so.0 => /usr/lib/x86_64-linux-gnu/libxcb-dri3.so.0 (0x00007f038780d000)
libxcb-present.so.0 => /usr/lib/x86_64-linux-gnu/libxcb-present.so.0 (0x00007f038760a000)
libxcb-sync.so.1 => /usr/lib/x86_64-linux-gnu/libxcb-sync.so.1 (0x00007f0387403000)
libxshmfence.so.1 => /usr/lib/x86_64-linux-gnu/libxshmfence.so.1 (0x00007f0387200000)
libglapi.so.0 => /usr/lib/x86_64-linux-gnu/libglapi.so.0 (0x00007f0386fcf000)
libXext.so.6 => /usr/lib/x86_64-linux-gnu/libXext.so.6 (0x00007f0386dbd000)
libXdamage.so.1 => /usr/lib/x86_64-linux-gnu/libXdamage.so.1 (0x00007f0386bba000)
libXfixes.so.3 => /usr/lib/x86_64-linux-gnu/libXfixes.so.3 (0x00007f03869b4000)
libX11-xcb.so.1 => /usr/lib/x86_64-linux-gnu/libX11-xcb.so.1 (0x00007f03867b2000)
libxcb-glx.so.0 => /usr/lib/x86_64-linux-gnu/libxcb-glx.so.0 (0x00007f0386599000)
libxcb-dri2.so.0 => /usr/lib/x86_64-linux-gnu/libxcb-dri2.so.0 (0x00007f0386394000)
libxcb.so.1 => /usr/lib/x86_64-linux-gnu/libxcb.so.1 (0x00007f0386172000)
libdrm.so.2 => /usr/lib/x86_64-linux-gnu/libdrm.so.2 (0x00007f0385f61000)
libpthread.so.0 => /lib/x86_64-linux-gnu/libpthread.so.0 (0x00007f0385d44000)
libdl.so.2 => /lib/x86_64-linux-gnu/libdl.so.2 (0x00007f0385b40000)
/lib64/ld-linux-x86-64.so.2 (0x00007f0389132000)
libXau.so.6 => /usr/lib/x86_64-linux-gnu/libXau.so.6 (0x00007f038593c000)
libXdmcp.so.6 => /usr/lib/x86_64-linux-gnu/libXdmcp.so.6 (0x00007f0385736000)
```
- glxinfo
```sh
# glxinfo | more
libGL error: No matching fbConfigs or visuals found
libGL error: failed to load driver: swrast
Error: couldn't find RGB GLX visual or fbconfig
name of display: :0
132 GLX Visuals
visual x bf lv rg d st colorbuffer sr ax dp st accumbuffer ms cav
id dep cl sp sz l ci b ro r g b a F gb bf th cl r g b a ns b eat
```
# Solution
## Dockerfile
- Use nvidia/cudagl rather than nvidia/cuda
```sh
# versiion
ARG cuda_version=9.0
# ARG cudnn_version=7
ARG ubuntu_version=16.04
ARG nvidia_cudnn_version=7.1.3.16-1+cuda9.0
FROM nvidia/cudagl:${cuda_version}-devel-ubuntu${ubuntu_version}
# FROM nvidia/cuda:${cuda_version}-cudnn${cudnn_version}-devel-ubuntu16.04
# base image
MAINTAINER [email protected]
# ENV LANG C.UTF-8
# ENV LC_ALL C.UTF-8
################################################################################
# Install the Nvidia cuDNN library missing in the parent image.
# https://gitlab.com/nvidia/cuda/blob/ubuntu16.04/10.1/devel/cudnn7/Dockerfile
ARG nvidia_cudnn_version
ENV NVIDIA_CUDNN_VERSION=${nvidia_cudnn_version}
RUN apt-get update && apt-get install -y --no-install-recommends \
libcudnn7=${NVIDIA_CUDNN_VERSION} \
libcudnn7-dev=${NVIDIA_CUDNN_VERSION} \
&& apt-mark hold libcudnn7 \
&& rm -rf /var/lib/apt/lists/*
################################################################################
# Install ROS Kinetic Kame.
# http://wiki.ros.org/kinetic/Installation/Ubuntu
# Update the package list.
RUN echo "deb http://packages.ros.org/ros/ubuntu xenial main" > /etc/apt/sources.list.d/ros-latest.list
# Add the package keys.
RUN apt-key adv --keyserver 'hkp://ha.pool.sks-keyservers.net:80' --recv-key C1CF6E31E6BADE8868B172B4F42ED6FBAB17C654
# Install 'ros-kinetic-desktop-full' packages (including ROS, Rqt, Rviz, and more).
#ARG ros_desktop_version
#ENV ROS_DESKTOP_VERSION=${ros_desktop_version}
RUN apt-get update && apt-get install -y --no-install-recommends \
ros-kinetic-desktop-full \
&& rm -rf /var/lib/apt/lists/*
# Install ROS bootstrap tools
RUN apt-get update && apt-get install --no-install-recommends -y \
python-rosdep \
python-rosinstall \
python-vcstools \
python-rosinstall-generator \
python-wstool
RUN rosdep init \
&& rosdep update
################################################################################
```
## Docker-compose
- Add
```sh
runtime: nvidia
environment:
- DISPLAY
- QT_X11_NO_MITSHM=1
volumes:
- /tmp/.X11-unix:/tmp/.X11-unix:rw
```
Sample:
```sh
version: '2.3'
services:
ubuntu16_cuda9_ros:
image: yubaoliu/root:ros-cuda9.0-cudnn7-ubuntu16.04
build:
context: .
dockerfile: Dockerfile
args:
cuda_version: 9.0
# cudnn_version: 7
ubuntu_version: 16.04
runtime: nvidia
stdin_open: true
tty: true
environment:
- DISPLAY
- QT_X11_NO_MITSHM=1
volumes:
- /tmp/.X11-unix:/tmp/.X11-unix:rw
- ubuntu16_cuda9_ros:/root
# entrypoint: /entrypoint-kinetic.sh
volumes:
ubuntu16_cuda9_ros:
```
## xhost +local:root
# Verify
```sh
xhost +local:root
docker-compose -f ros_cuda9.0.yml up
Start a terminal
roscore
```
```sh
# rosrun rviz rviz
[ INFO] [1591758684.872139458]: rviz version 1.12.17
[ INFO] [1591758684.872168343]: compiled against Qt version 5.5.1
[ INFO] [1591758684.872175509]: compiled against OGRE version 1.9.0 (Ghadamon)
process 107: D-Bus library appears to be incorrectly set up; failed to read machine uuid: UUID file '/etc/machine-id' should contain a hex string of length 32, not length 0, with no other text
See the manual page for dbus-uuidgen to correct this issue.
[ INFO] [1591758685.537067433]: Stereo is NOT SUPPORTED
[ INFO] [1591758685.537229733]: OpenGl version: 4.6 (GLSL 4.6).
```
# References
- [How to Install OpenGL on Ubuntu Linux](http://www.codebind.com/linux-tutorials/install-opengl-ubuntu-linux/)
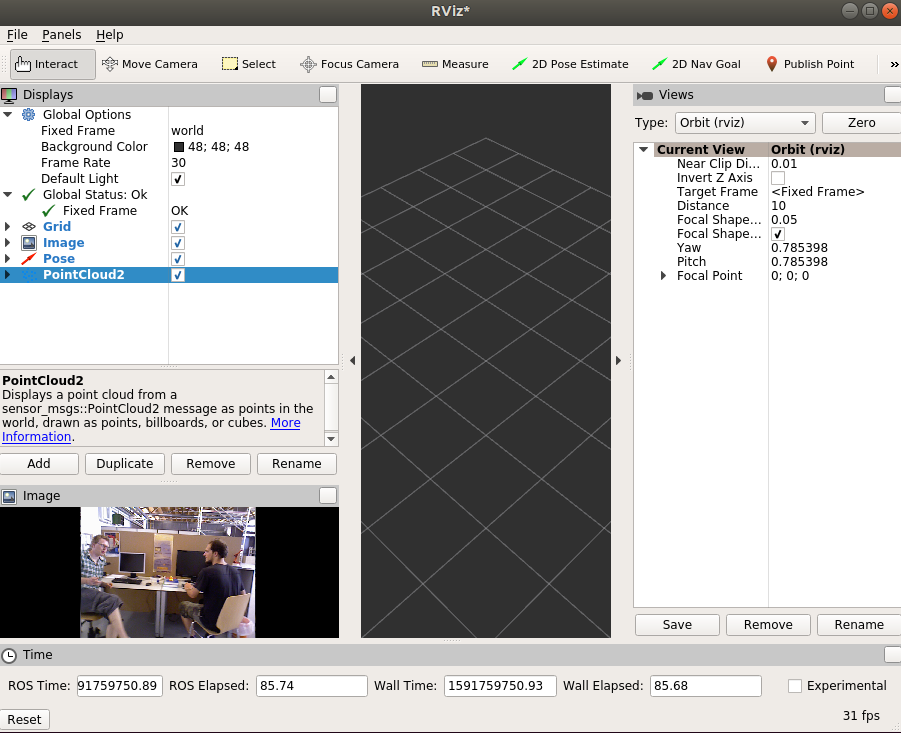
No comments