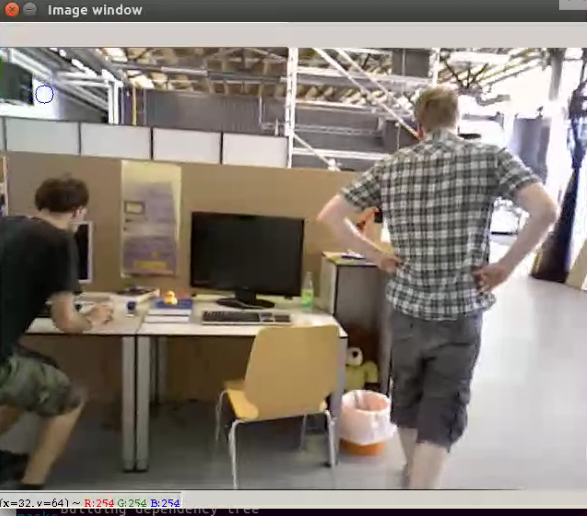
Conversion Between ROS imgmsg and OpenCV: cv_bridge Example (Python)
# Synopsis
- 如何在OpenCV与ROS图像消息之间进行转换
- [Converting between ROS images and OpenCV images (Python)](http://wiki.ros.org/cv_bridge/Tutorials/ConvertingBetweenROSImagesAndOpenCVImagesPython)
# Create Package
```sh
catkin_create_pkg cvbridge_example sensor_msgs cv_bridge rospy std_msgs
```
Note that:
Please do not add "opencv2" here.
# Converting ROS image messages to OpenCV images
```python
from from cv_bridge import CvBridge
bridge = CvBridge()
cv_image = bridge.imgmsg_to_cv2(image_message, desired_encoding='passthrough')
```
# Converting OpenCV images to ROS image messages
```python
from cv_bridge import CvBridge
bridge = CvBridge()
image_message = bridge.cv2_to_imgmsg(cv_image, encoding="passthrough")
```
# Python Exampe
```python
#!/usr/bin/env python
from __future__ import print_function
import roslib
roslib.load_manifest('cvbridge_example')
import sys
import rospy
import cv2
from std_msgs.msg import String
from sensor_msgs.msg import Image
from cv_bridge import CvBridge, CvBridgeError
class image_converter:
def __init__(self):
self.image_pub = rospy.Publisher("result_image",Image)
self.bridge = CvBridge()
self.image_sub = rospy.Subscriber("/camera/rgb/image_color",Image,self.callback)
def callback(self,data):
try:
cv_image = self.bridge.imgmsg_to_cv2(data, "bgr8")
except CvBridgeError as e:
print(e)
(rows,cols,channels) = cv_image.shape
if cols > 60 and rows > 60 :
cv2.circle(cv_image, (50,50), 10, 255)
cv2.imshow("Image window", cv_image)
cv2.waitKey(3)
try:
self.image_pub.publish(self.bridge.cv2_to_imgmsg(cv_image, "bgr8"))
except CvBridgeError as e:
print(e)
def main(args):
ic = image_converter()
rospy.init_node('image_converter', anonymous=True)
try:
rospy.spin()
except KeyboardInterrupt:
print("Shutting down")
cv2.destroyAllWindows()
if __name__ == '__main__':
main(sys.argv)
```
# Run example
```sh
chmod +x example.py
rosrun cvbridge_example example.py
```
Play bag file manually. For example, TUM bag file.
# Result
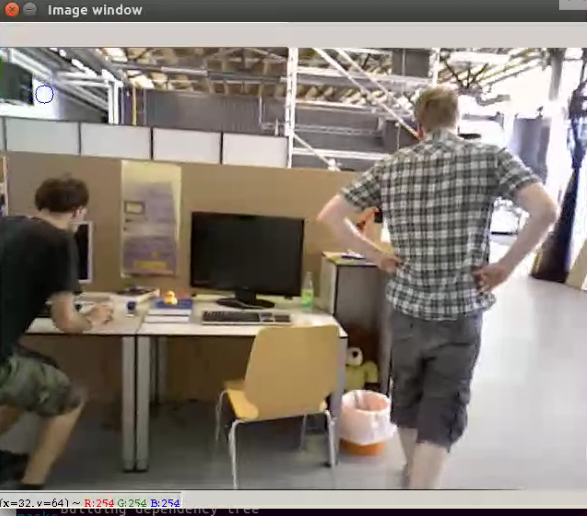
No comments